Best Practices for Secure Programming in Rust
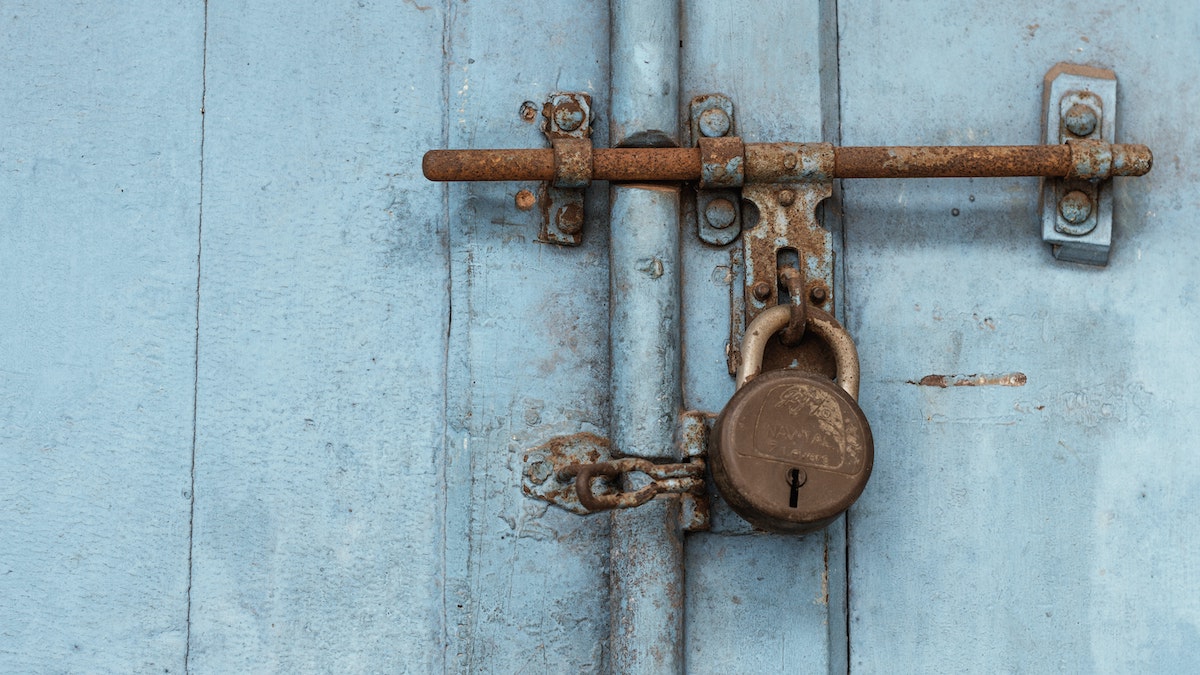
Rust is a modern programming language that is known for its safety and security features. As a Rust developer, you understand the importance of writing secure code. Rust's memory safety and type system help prevent entire classes of vulnerabilities, but that doesn't mean Rust's code is impervious to security issues. There are still risks from logic errors, improper handling of edge cases, and malicious inputs that you must consider.
Thus, the objective of this article is to provide you—Rust developers—with some best practices and recommendations for secure application development. These best practices will enable you to take advantage of the range of security possibilities and features that Rust has to offer.
By following these essential security best practices, you can harden your Rust applications and minimize the attack surface while giving your users the safe, robust experience they deserve.
Common Security Threats in Rust Applications
As a Rust developer, you must be aware of common security threats faced by Rust applications.
Some of the major threats include:
- Buffer overflows: Although Rust's ownership and borrowing constraints prevent many memory safety issues, unsafe code can still cause vulnerabilities such as buffer overflows or dangling pointers. When more data is written to a buffer than it can hold, the buffer overflows. This can result in memory corruption, which attackers might leverage to take control of the program.
- Injection attacks: Injection attacks occur when untrusted data is injected into a program, such as through a SQL query or a command line argument. This can lead to data loss, unauthorized access, or other security problems.
- Race conditions: Race conditions occur when two or more threads of execution are accessing the same data at the same time. This can lead to data corruption or other problems.
- Cryptographic weaknesses: Poor use of cryptographic primitives can compromise data confidentiality and integrity. Cryptographic weaknesses are also caused by the use of untrusted crates for cryptography and failure to follow best practices, leading to data integrity corruption.
{{code-cta}}
Best Practices for Secure Rust Coding
To write secure Rust code, there are several best practices you should follow:
1. Use Rust’s Type System to Your Advantage
Rust’s strict type system helps prevent invalid memory access, null pointer dereferences, and type confusion. Define types as specifically as possible and avoid using Any or dyn Trait unless absolutely necessary.
2. Sanitize Input Data
Audit all input data and sanitize it thoroughly before it is used by the Rust program. You can achieve this by carefully validating the user input data and sanitizing it appropriately before use to prevent injection vulnerabilities such as SQL injection or cross-site scripting.
3. Keep Dependencies Up to Date
Use the latest versions of dependencies to ensure you have the most recent security patches and fixes. Monitor dependencies for new releases regularly.
4. Minimize the Use of Unsafe Blocks
Unsafe blocks in Rust allow developers to bypass the type system and memory management system. Although this can be useful for performance reasons, it can also introduce security vulnerabilities.
For example, if a developer uses an unsafe block to access a memory location that is not owned by the current thread, this can lead to a race condition. A race condition is a condition where two or more threads are accessing the same data at the same time, and the outcome of the program depends on the order in which the threads access the data. Race conditions can be exploited by attackers to gain control of the program.
Another security vulnerability that can be introduced by unsafe blocks is buffer overflows, which might lead to memory corruption and can be exploited by attackers to gain control of the program.
For these reasons, it is important to minimize the use of unsafe blocks in Rust. You must always review any unsafe code thoroughly to ensure there is no undefined behavior.
5. Use Cryptography Carefully
Rust provides several secure algorithms, including cryptographic algorithms. Cryptography is easy to implement incorrectly. As a developer, you ought to ensure that you use proven, audited crates and that you review cryptographic code meticulously to protect sensitive data.
6. Use Rust’s Built-In Security Mitigations
Features like stack canaries, ASLR, and overflow checks help to prevent common vulnerabilities and security problems like buffer overflows and race conditions. You should not disable these features.
Leveraging Rust's Security Features
Rust has several built-in features that enable secure coding practices. You should utilize these features to strengthen your applications.
Here are some of the security features that Rust provides:
- Ownership and borrowing: Rust's ownership and borrowing rules ensure memory safety and prevent vulnerabilities like buffer overflows. You must follow these rules carefully in your code.
- Static typing system: Rust's strict static typing prevents type confusion issues that lead to vulnerabilities in other languages. You must annotate all variables and functions with concrete types to leverage this feature.
- Pattern matching: Rust's pattern matching can be used to ensure that only valid data is handled in your code. You should use pattern matching (especially on external inputs) to validate data and handle invalid data appropriately.
- Sandboxing: Rust supports sandboxing, which can help to isolate malicious code.
- Minimal runtime: Rust's minimal runtime reduces the attack surface for your applications. You should avoid using unsafe blocks and external crates whenever possible to maintain this minimal runtime.
- Cryptographic algorithms: Rust provides several cryptographic algorithms, such as AES and SHA-256. These algorithms can be used to protect sensitive data.
Examples of Secure Coding Techniques in Rust
To write secure Rust code, you should employ techniques that minimize vulnerabilities – including the following:
1. Use Functions
Functions can help to encapsulate code and make it easier to reason. This can help prevent vulnerabilities. For example, the following function written in Rust checks whether a given string is a valid email address or not:
2. Use Modules
Modules can help to organize code and make it easier to understand. This can help prevent vulnerabilities. The following code, for example, depicts a module containing functions for performing common security tasks such as sanitizing input data and encrypting data:
3. Use Tests
Tests can help find and fix bugs in code. This can help prevent vulnerabilities. Here is an example of how you can write tests in Rust securely to catch any authentication-authorization anomalies:
Conclusion
Rust is a powerful programming language that can be used to write secure applications. Rust's memory safety and type system provide a strong foundation, but secure programming requires diligence and proactive thinking about potential vulnerabilities.
By making security a priority in your development process and staying vigilant for new threats, you can leverage Rust to build software that stands the test of time. The future is bright for secure systems development in Rust.
Add Mayhem to Your DevSecOps for Free.
Get a full-featured 30 day free trial.
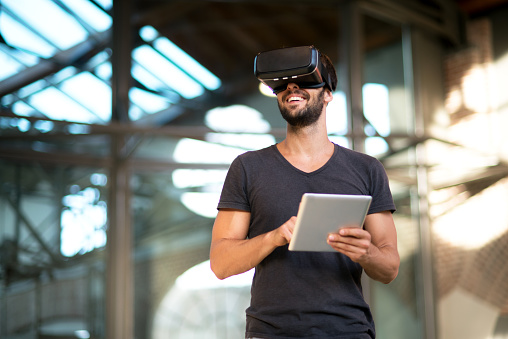